일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- parse
- querydsl no sources given
- springboot
- java 1.8 11
- JUnit
- AWS CLI
- no sources given
- OpenFeign
- xcrun: error: invalid active developer path (/Library/Developer/CommandLineTools)
- java version
- yum install java
- aws
- error
- maybe not public or not valid?
- Java 1.8
- java 여러개 버전
- property or field 'jobparameters' cannot be found on object of type
- No tests found for given includes
- springbatch error
- easy
- java 11
- springboottest
- Medium
- mac os git error
- 스프링부트테스트
- log error
- java 버전 변경
- el1008e
- LeetCode
- java
- Today
- Total
쩨이엠 개발 블로그
[ Leetcode ] 1669. Merge In Between Linked Lists - Java 본문
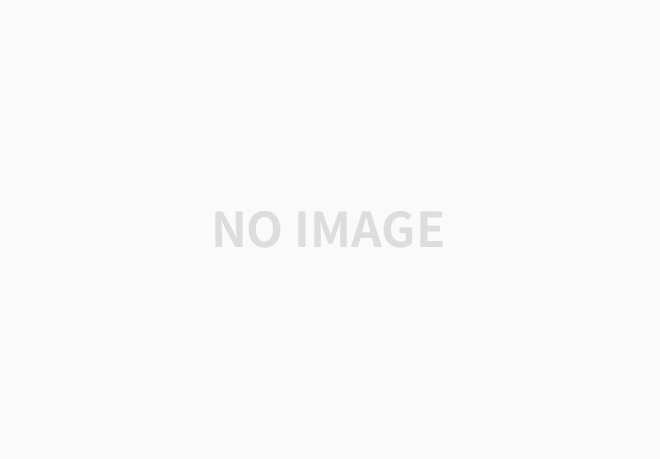
You are given two linked lists: list1 and list2 of sizes n and m respectively.
Remove list1's nodes from the ath node to the bth node, and put list2 in their place.
The blue edges and nodes in the following figure incidate the result:
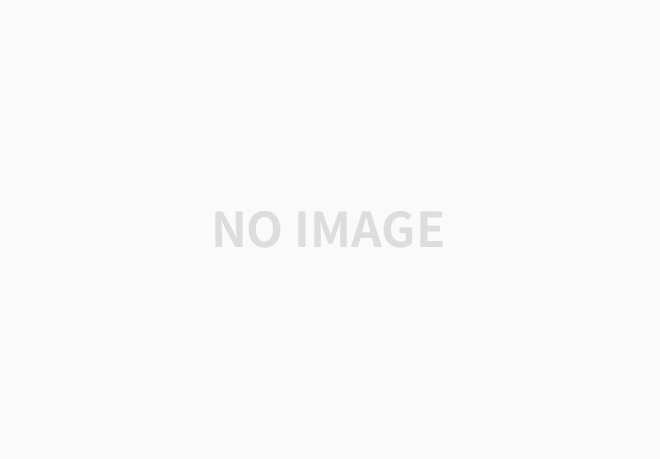
Build the result list and return its head.
Example 1:
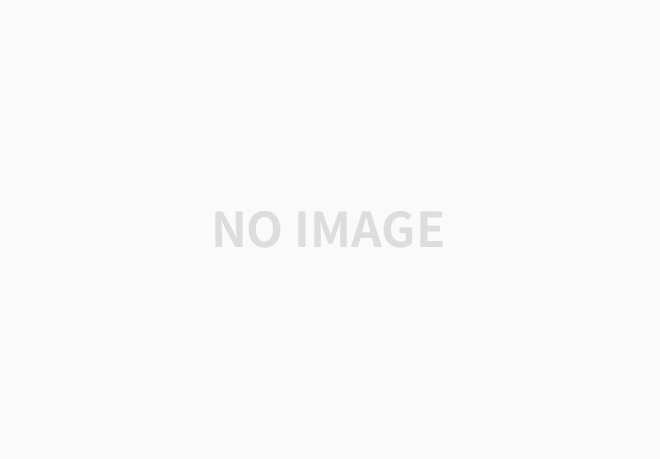
Input: list1 = [0,1,2,3,4,5], a = 3, b = 4, list2 = [1000000,1000001,1000002]
Output: [0,1,2,1000000,1000001,1000002,5]
Explanation: We remove the nodes 3 and 4 and put the entire list2 in their place.
The blue edges and nodes in the above figure indicate the result.
Example 2:
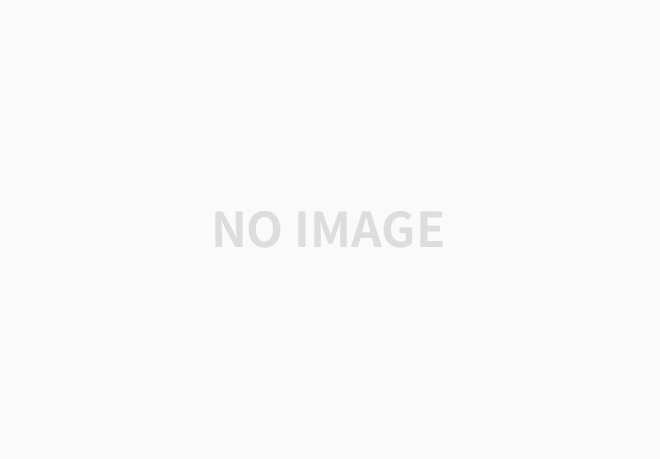
Input: list1 = [0,1,2,3,4,5,6], a = 2, b = 5, list2 = [1000000,1000001,1000002,1000003,1000004]
Output: [0,1,1000000,1000001,1000002,1000003,1000004,6]
Explanation: The blue edges and nodes in the above figure indicate the result.
Constraints:
- 3 <= list1.length <= 104
- 1 <= a <= b < list1.length - 1
- 1 <= list2.length <= 104
Solution
import java.util.*;
class Solution {
public ListNode mergeInBetween(ListNode list1, int a, int b, ListNode list2) {
List<Integer> list = getValueList(list1);
int end = list.get(list.size()-1);
ListNode answer = new ListNode(list.get(end));
for(int i=end-1; i> b; i--){
answer = new ListNode(list.get(i), answer);
}
int max = getMax(list2);
for(int i=max; i>= 1000000; i--){
answer = new ListNode(i, answer);
}
for(int i=a-1; i >= 0; i--){
answer = new ListNode(list.get(i), answer);
}
return answer;
}
public int getMax(ListNode list){
int max = 0;
while(list != null){
if(list.next == null){
max = list.val;
}
list = list.next;
}
return max;
}
public List<Integer> getValueList(ListNode list){
List<Integer> arrList = new ArrayList<>();
while(list != null){
arrList.add(list.val);
list = list.next;
}
return arrList;
}
}
1. ListNode 값을 ArrayList에 담는다
2. 마지막 값에서 b번째 전까지의 값으로 ListNode를 만들다
3. 껴넣을 ListNode도 마지막 값부터 ListNode로 만들어준다 -> 이부분을 한번에 하면 좋을것같은데 아직 모르겠다
4. 남은 0번째부터 a번째까지의 값도 ListNode로 만든다
너무 느리다 방법 다시 찾기 (++추가!)
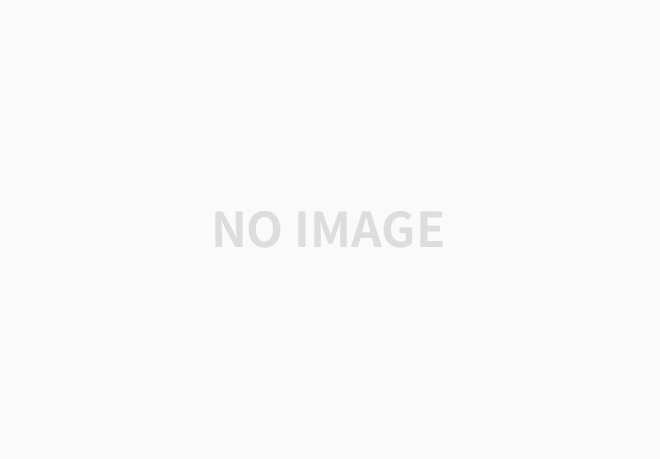
++ 앞뒤 ListNode를 구분지어보기로 한다
public class Solution {
public ListNode mergeInBetween(ListNode list1, int a, int b, ListNode list2) {
ListNode temp = new ListNode(0);
temp.next = list1;
ListNode front = temp;
ListNode back = temp;
for(int i = 0; i < a; i++){
front = front.next;
}
front.next = list2;
while(list2.next != null){
list2 = list2.next;
}
for(int i = 0; i <= b; i++){
back = back.next;
}
list2.next = back.next;
return temp.next;
}
}
1. 시작 노드부터 next로 a번째까지 간 뒤 머지할 list2를 넣어준다
2. list2도 계속 뒤로뒤로 해서 끝까지 간다
3. 시작 노드부터 next로 b번째까지 간 뒤 list2 뒤에 붙여준다
4. list1을 시작한 temp.next를 return 한다
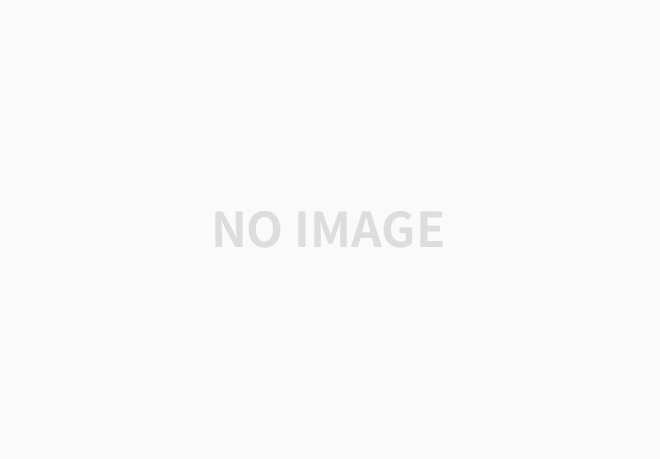
100%!!!
근데 여전히 헷갈리는 중..... 비슷한 문제를 좀 더 풀어봐야겠다
'개발 > Programming' 카테고리의 다른 글
[ Leetcode ] 9. Palindrome Number - Java (0) | 2021.03.08 |
---|---|
[ Leetcode ] 6. ZigZag Conversion - Java (0) | 2021.02.25 |
[ Leetcode ] 148. Sort List - Java (0) | 2021.02.10 |
[ Leetcode ] 324. Wiggle Sort II - Java (0) | 2021.02.08 |
[ Leetcode ] 1561. Maximum Number of Coins You Can Get - Java (0) | 2021.02.07 |